mirror of
https://github.com/ProjectSynthoria/SynthoriaArchive.git
synced 2025-03-12 15:26:56 +02:00
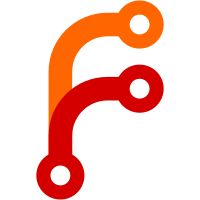
* Fix total torrent count for user listings The total count would previously be bound by the maximum number of pages we allow. Since we run the count query anyway, we can just save this result and use it in the template. * search: allow users to view all their uploads Maximum page limitations shouldn't take effect when users are looking at a listing of their own torrents.
62 lines
1.8 KiB
Python
62 lines
1.8 KiB
Python
import os.path
|
|
|
|
from flask import abort
|
|
from flask.config import Config
|
|
from flask_assets import Environment
|
|
from flask_caching import Cache
|
|
from flask_debugtoolbar import DebugToolbarExtension
|
|
from flask_sqlalchemy import BaseQuery, Pagination, SQLAlchemy
|
|
|
|
assets = Environment()
|
|
db = SQLAlchemy()
|
|
toolbar = DebugToolbarExtension()
|
|
cache = Cache(config={'CACHE_TYPE': 'simple'})
|
|
|
|
|
|
class LimitedPagination(Pagination):
|
|
def __init__(self, actual_count, *args, **kwargs):
|
|
self.actual_count = actual_count
|
|
super().__init__(*args, **kwargs)
|
|
|
|
|
|
def fix_paginate():
|
|
|
|
def paginate_faste(self, page=1, per_page=50, max_page=None, step=5, count_query=None):
|
|
if page < 1:
|
|
abort(404)
|
|
|
|
if max_page and page > max_page:
|
|
abort(404)
|
|
|
|
# Count all items
|
|
if count_query is not None:
|
|
total_query_count = count_query.scalar()
|
|
else:
|
|
total_query_count = self.count()
|
|
actual_query_count = total_query_count
|
|
if max_page:
|
|
total_query_count = min(total_query_count, max_page * per_page)
|
|
|
|
# Grab items on current page
|
|
items = self.limit(per_page).offset((page - 1) * per_page).all()
|
|
|
|
if not items and page != 1:
|
|
abort(404)
|
|
|
|
return LimitedPagination(actual_query_count, self, page, per_page, total_query_count,
|
|
items)
|
|
|
|
BaseQuery.paginate_faste = paginate_faste
|
|
|
|
|
|
def _get_config():
|
|
# Workaround to get an available config object before the app is initiallized
|
|
# Only needed/used in top-level and class statements
|
|
# https://stackoverflow.com/a/18138250/7597273
|
|
root_path = os.path.abspath(os.path.join(os.path.dirname(__file__), '..'))
|
|
config = Config(root_path)
|
|
config.from_object('config')
|
|
return config
|
|
|
|
|
|
config = _get_config()
|